JBang Meets Spring Boot & LangChain4j: A Powerhouse for Java Scripting and AI Pipelines
When was the last time you used Java in a pipeline script? Today, we'll explore how to use Java for scripting by building a tooling script for this blog.
I was waiting for a good use case for JBang, and then I realized it's not limited to simple single-file use cases. You can actually bring along Spring Boot, and usually, that's more than enough for me. Just give me that Spring context, and I'm good to go.
Use Case
I don't always have time to be meticulous in the articles I write here, as I am building everything from scratch. In this case, you are both the developer and the reporter.
I think it's good to leverage AI, learn scripting with Java through JBang, and explore the possibilities of LangChain4j, which was the driver for this article.
The plan is to run inferences against the Markdown files in this blog using DeepSeek while respecting the original Markdown structure and the author's intent.
AI, when used to augment your capabilities, is wonderful. It opens the door to a perpetually improving machine that doesn't tire.
I'll share some of the PR suggestions that DeepSeek came up with for the articles shared here.
Remember, you are in control. You can always choose to ignore the suggestions.
JBang
Introduction
JBang has done a lot for Java when it comes to quickly bootstrapping a project in a minute or less. It's a great tool for scripting in Java.
Although I believe I am slightly abusing the original intent of the tool by bringing in Spring Boot and packaging this script as a complete project, I am happy with the power this brings to the table. I can see Java in CI/CD pipelines in the future as a valid option that brings type safety and the rich standard API that Java is known for in a traditional pipeline.
What a time to be alive: NIO 2, Streams, in what traditionally was a bash script.
My understanding is that this can shift the general feeling that pipelines are second-class citizens. Once they are established, we rarely venture to improve them. In your project, this can power what I would call Pipelines As Code (PaC?).
Getting Started
As mentioned before, JBang aims to get you started with Java rather quickly:
This will create a script that you can run with JBang:
I didn't want to cram everything into a single file, and JBang has support for multi-source files, so I went with that.
Let's explore how to set up a project with JBang, IntelliJ, and Spring here.
Since this blog is not a Java project, I am creating a folder to hold the future scripts:
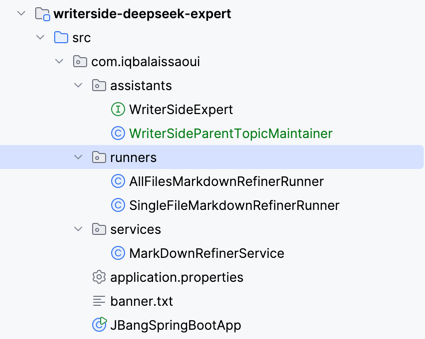
To get full support from IntelliJ, you'll have to create a module this time since this is a regular folder and not a Maven project.
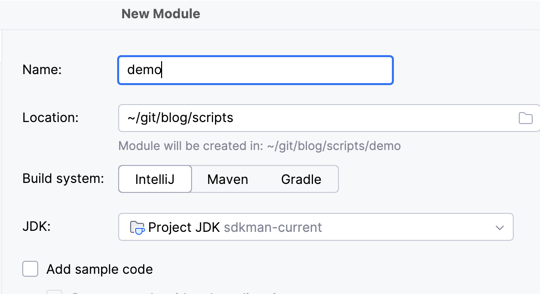
You can initialize JBang within the src
folder and use package names as you would in a regular Java project.
There is also a JBang plugin for IntelliJ that you'll need for a better experience.
Worth noting that the dependency imports need you to run this action to be added to the project module.
You can run it on the entry point file JBangSpringBootApp.java
.
Walkthrough the Code
Let's go through the files you see in the tree above:
Starting with the entry point JBangSpringBootApp.java
:
//DEPS
are the dependencies that JBang will download for you, and you can use them in your script.//FILES
are the files that JBang will include in the build. We are using it here for Spring Boot's typicalapplication.properties
andbanner.txt
.//SOURCES
are the source files JBang will compile and include in the build.
We will be using:
Directly the Spring Boot starter as we don't need the embedded web server.
LangChain4j Spring integration for its easy-to-bootstrap high-level API for AI inference (AI Services).
Command Line Runners loaded conditionally by profiles for two use cases: all files in the blog and a single file.
The AI interface leveraging LangChain4j is in WriterSideExpert.java
:
MarkDownRefinerService.java
is the service that will use the AI interface to refine the Markdown files:
Let's take a look at the runners next:
SingleFileMarkdownRefinerRunner.java
to target a single Markdown file for improvements:
The rich standard API of Java here shines:
We can use the Files
class from NIO 2 to easily read and write files, and StringUtils
from Apache Commons to compare the differences between the original and the improved Markdown.
We will also leverage the Stream API in the all-files processor.
AllFilesMarkdownRefinerRunner.java
:
This runner reads all Markdown files that belong to the specific WriterSide instance (hi.tree
filter) and processes them with the AI service.
Finally, let's go through the application.properties
:
I am only using these properties:
In particular, it's worth mentioning that the default timeout was not enough for some of the inferences, so I had to increase it.
Running the Script
Simply run the script with JBang from the same folder where the entry point file is (this is from within com.iqbalaissaoui
):
For the single file runner, pass the file name as an argument (without the path as I included a base folder in the runner):
For the all files runner:
And that's it! Here's a sneak peek at the suggested output:
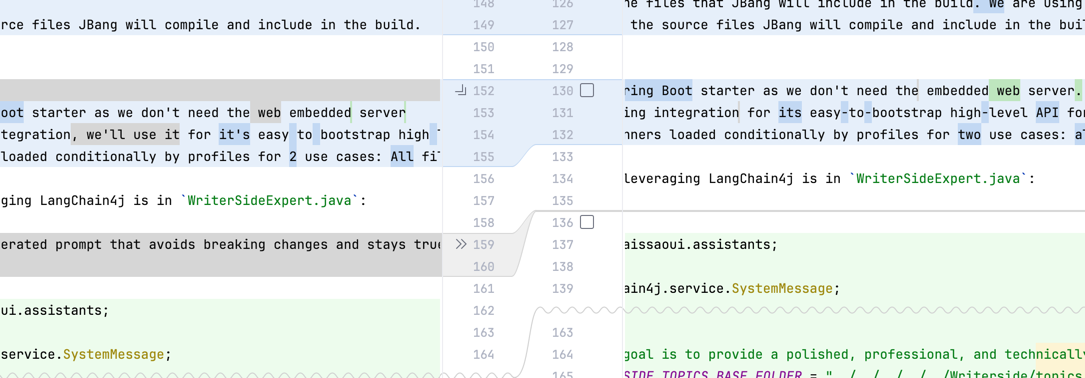
Source Code
The source code for the writerside_experts
module discussed in this article can be found in the following public repository:
Where to Go from Here
Now the sky is the limit. You can chain AI Services that handle different scenarios with Java's UnaryOperator to keep the setup simple: